In this article and the next one, I will demonstrate some common operations on List<string> using LINQ. I assume that you are familiar with LINQ.
Here are the sample List<string> for demonstration purposes. The VB.NET code has been converted using a conversion tool and accuracy has not been tested.
C#
List<string> lstOne = new List<string>() { "January", "February", "March"};
List<string> lstTwo = new List<string>() { "January", "April", "March"};
List<string> lstThree = new List<string>() { "January", "April", "March", "May" };
List<string> lstFour = new List<string>() { "Jan", "Feb", "Jan", "April", "Feb" };
IEnumerable<string> lstNew = null;
VB.NET
Dim lstOne As New List(Of String) (New String() {"January", "February", "March"})
Dim lstTwo As New List(Of String) (New String() {"January", "April", "March"})
Dim lstThree As New List(Of String) (New String() {"January", "April", "March", "May"})
Dim lstFour As New List(Of String) (New String() {"Jan", "Feb", "Jan", "April", "Feb"})
Dim lstNew As IEnumerable(Of String) = Nothing
We will be printing the results on the console using a simple method shown here:
C#
static void PrintList(IEnumerable<string> str)
{
foreach (var s in str)
Console.WriteLine(s);
Console.WriteLine("-------------");
}
VB.NET
Shared Sub PrintList(ByVal str As IEnumerable(Of String))
For Each s In str
Console.WriteLine(s)
Next s
Console.WriteLine("-------------")
End Sub
Let us get started.
1. Display common elements between two List<string>
C#
// Compare two List<string> and display common elements
lstNew = lstOne.Intersect(lstTwo, StringComparer.OrdinalIgnoreCase);
PrintList(lstNew);
VB.NET
lstNew = lstOne.Intersect(lstTwo, StringComparer.OrdinalIgnoreCase)
PrintList(lstNew)
OUTPUT
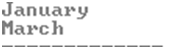
2. Display elements found in one List<string> but not in the other
C#
// Compare two List<string> and display items of lstOne not in lstTwo
lstNew = lstOne.Except(lstTwo, StringComparer.OrdinalIgnoreCase);
PrintList(lstNew);
VB.NET
lstNew = lstOne.Except(lstTwo, StringComparer.OrdinalIgnoreCase)
PrintList(lstNew)
Note: The StringComparer.OrdinalIgnoreCase performs a case-insensitive ordinal string comparison
OUTPUT
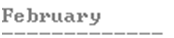
3. Display distinct elements from a List<string>
C#
// Unique List<string>
lstNew = lstFour.Distinct(StringComparer.OrdinalIgnoreCase);
PrintList(lstNew)
VB.NET
lstNew = lstFour.Distinct(StringComparer.OrdinalIgnoreCase)
PrintList(lstNew)
OUTPUT
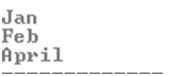
4. Convert all elements of a List<string> to UpperCase
C#
// Convert elements of List<string> to Upper Case
lstNew = lstOne.ConvertAll(x => x.ToUpper());
PrintList(lstNew);
VB.NET
lstNew = lstOne.ConvertAll(Function(x) x.ToUpper())
PrintList(lstNew)
OUTPUT
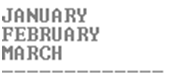
5. Concatenate and Sort two List<string>
C#
// Concatenate and Sort two List<string>
lstNew = lstOne.Concat(lstTwo).OrderBy(s => s);
PrintList(lstNew);
VB.NET
lstNew = lstOne.Concat(lstTwo).OrderBy(Function(s) s)
PrintList(lstNew)
OUTPUT
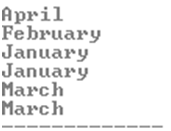
6. Concatenate Unique Elements of two List<string>
C#
// Concatenate Unique Elements of two List<string>
lstNew = lstOne.Concat(lstTwo).Distinct();
PrintList(lstNew);
VB.NET
lstNew = lstOne.Concat(lstTwo).Distinct()
PrintList(lstNew)
OUTPUT
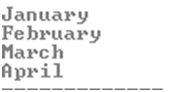
7. Reverse a List<string>
C#
// Reverse a List<string>
lstOne.Reverse();
PrintList(lstOne);
VB.NET
lstOne.Reverse()
PrintList(lstOne)
OUTPUT
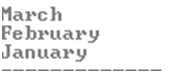
8. Search a List<string> and Remove the Search Item from the List<string>
C#
// Search a List<string> and Remove the Search Item
// from the List<string>
int cnt = lstFour.RemoveAll(x => x.Contains("Feb"));
Console.WriteLine("{0} items removed", cnt);
PrintList(lstFour);
VB.NET
Dim cnt As Integer = lstFour.RemoveAll(Function(x) x.Contains("Feb"))
Console.WriteLine("{0} items removed", cnt)
PrintList(lstFour)
OUTPUT
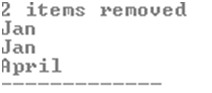